The Microsoft ReportViewer control is a powerfull control for embedding reports in a .Net application. There are two versions of this control: a WinForms version and a WebForms (Asp.Net) version. Unfortunately there is no native WPF version available at this moment but it is possible to use this control in a WPF application as explained later in this post.
The ReportViewer control has a number of advantages:
– is a freely redistributable component
– is able to show RDLC (Report Definition Language Client-side) format reports when the control is in local mode
– is able to show RDL ((Report Definition Language) format reports from a SQL Service Reporting Service (SSRS) when the control is in remote mode
– can export reports to Excel, PDF and Word (and additional formats when running in remote mode) without having Excel, Adobe Reader or Word installed
– supports print preview
In this post I’am going to show how to:
– use the ReportViewer control in a WPF application
– use an OData service as a datasource for the report
– use a report with a sub report
Using the ReportViewer control in a WPF application
As mentioned before the ReportViewer control is not available as a native WPF control but only as a WinForms and WebForms control. With the WPF WindowsFormsHost control is possible to host WinForms controls in a WPF application so also the WinForms ReportViewer control.
To use the WindowsFormsHost control together with the WinForms ReportViewer control you must add references to the following assemblies:
– WindowsFormsIntegration
– System.Windows.Forms
– Microsoft.ReportViewer.WinForms
After adding the references you can use the ReportViewer control in XAML.
Using an OData service as datasource
The RDLC reports can use many different sources as datasource. In this post I’am using the public available Northwind OData service as datasource. To use this service add a service reference in a Visual Studio project (in my case a WPF application) to http://services.odata.org/Northwind/Northwind.svc.To retrieve data from this datasource I created a Repository class which is responsible for communicating with the service.
public static class Repository
{
public static IEnumerable GetCustomers()
{
return NorthwindEntitiesDataService.Customers;
}
public static IEnumerable GetOrdersForCustomer(string customerId)
{
return NorthwindEntitiesDataService.Orders.Where(o => o.CustomerID == customerId).Take(5);
}
private static NorthwindEntities _NorthwindEntitiesDataService;
private static NorthwindEntities NorthwindEntitiesDataService
{
get
{
if (_NorthwindEntitiesDataService == null)
{
_NorthwindEntitiesDataService = new NorthwindEntities(new Uri(@"http://services.odata.org/Northwind/Northwind.svc"));
}
return _NorthwindEntitiesDataService;
}
}
}
This Repository class will be used later in this post.
Report with a subreport
We will use two reports: a (main) report which shows customers (CustomerReport.rdlc) and a subreport (OrdersReport.rdlc) which shows the related orders. The CustomerReport uses the Customer dataset from the service reference as datasource and the OrdersReport will use the Customer (Orders) dataset as datasource.
In the subreport a parameter (CustomerIdParameter) is defined which is used for passing the customer Id from the CustomerReport to the OrdersReport (subreport) so the related orders can be retrieved and shown.
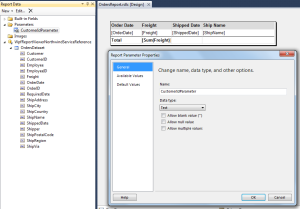
This parameter has to be filled in the CustomerReport. Therefore we have to specify in the properties of the subreport in the CustomerReport which field from the customer dataset is used for filling this parameter.
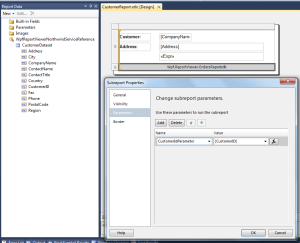
The reports are now ready to show the data. The last thing we have to do is telling the ReportViewer control to show this report. We already placed the ReportViewer control in a XAML file. In the code behind of the XAML file we have to add some code.
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
this.Loaded += new RoutedEventHandler(MainWindow_Loaded);
}
void MainWindow_Loaded(object sender, RoutedEventArgs e)
{
// get the customers from the OData service
IEnumerable customers = Repository.GetCustomers();
// get a reference to the WinForms ReportViewer control
Microsoft.Reporting.WinForms.ReportViewer reportViewer = (Microsoft.Reporting.WinForms.ReportViewer)windowsFormsHost.Child;
// subscribe to SubreportProcessing event for passing the related Orders data
reportViewer.LocalReport.SubreportProcessing += new SubreportProcessingEventHandler(LocalReport_SubreportProcessing);
// the report is included as an embedded resource in the assembly
// use the full name
reportViewer.LocalReport.ReportEmbeddedResource = "Wpf.ReportViewer.CustomerReport.rdlc";
// the CustomerReport has one dataset which must be filled
reportViewer.LocalReport.DataSources.Add(new ReportDataSource("CustomerDataset", customers));
// now let the ReportViewer render the report
reportViewer.RefreshReport();
}
void LocalReport_SubreportProcessing(object sender, SubreportProcessingEventArgs e)
{
// our subreport has only 1 parameter
string customerId = e.Parameters["CustomerIdParameter"].Values[0];
// our subreport has only 1 dataset
string dataSourceName = e.DataSourceNames[0];
// get the orders for the current customer
IEnumerable orders = Repository.GetOrdersForCustomer(customerId);
e.DataSources.Add(new ReportDataSource(dataSourceName, orders));
}
}
Because we are using a subreport we have to subscribe to the SubreportProcessing event of the ReportViewer. Each time a customer is rendered in the CustomerReport this event is fired. In the event arguments the value of the CustomerIdParameter is passed which we will use to retrieve the related orders from the OData service. After retrieving the orders data we fill the dataset for the subreport.
The Visual Studio 2010 solution can be downloaded from here